System¶
Authentication¶
-
POST
/api/v1/login
¶ Response JSON Object: - isAdmin (boolean) – is administrator group or not
- username (string) – request username
Example request (username and password)
$ curl -sq -XPOST -c cookies.txt -d '{"username": "admin", "password": "admin"}' \ http://${QIP}:${QPORT}/container-station/api/v1/login
Example request (sid)
$ curl -sq -XPOST -c cookies.txt -d '{"sid": "nassid"}' \ http://${QIP}:${QPORT}/container-station/api/v1/login
Example response
{ "anonymous": false, "isAdmin": true, "logintime": "2021-08-11 16:54:45", "username": "admin" }
Note
${QPORT} is the same as the system port of NAS.
Steps to check the system port: “Control Panel” > “General Settings”
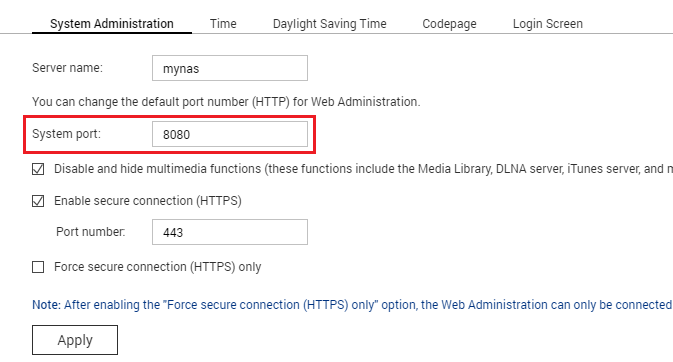
Note
Login API response will get HTTP 403 Forbidden status code if 2-step
verification is enabled on this account.
You should use sid as request body instead of username and password in login API.
-
GET
/api/v1/login_refresh
¶ Response JSON Object: - isAdmin (boolean) – is administrator group or not
- username (string) – request username
Example request
$ curl -sq -XGET -b cookies.txt http://${QIP}:${QPORT}/container-station/api/v1/login_refresh
Example response
{ "anonymous": false, "isAdmin": true, "logintime": "2021-08-11 16:54:45", "username": "admin" }
-
PUT
/api/v1/logout
¶ Response JSON Object: - username (string) – request username
Example request
$ curl -sq -XPUT -b cookies.txt http://${QIP}:${QPORT}/container-station/api/v1/logout
Example response
{ "username": "admin" }
System Information¶
-
GET
/api/v1/system
¶ Response JSON Object: - cpuCore (int) – CPU core count
- cpuThread (int) – Total CPU thread count
- hostname (string) – Device hostname
- processor (string) – Processor information
- machine (string) – Machine type, e.g. ‘x86_64’, ‘armv7l’. An empty string is returned if the value cannot be determined.
- version (object) – Version of Docker, LXC, LXD, and Container Station
- memoryPageSize (int) – Memory page size.
- features (object) – Support features, e.g. ‘lxd’, ‘net_bridge’
Example request
$ curl -sq -b cookies.txt http://${QIP}:${QPORT}/container-station/api/v1/system
Example response
{ "cpuCore": 4, "cpuThread": 4, "features": [ "", "net_bridge", "lxd" ], "gpu": { "csMode": false, "device": [], "driverInstalled": false }, "gpuchanged": false, "hostname": "SW8-ED-453Bm", "isDevicemapper": false, "isSupportOverlay": true, "machine": "amd64", "memory": 7998068, "memoryPageSize": 4096, "migrationDir": "/var/lib/docker/qnap-migration", "migrationDmStatus": "", "needRestart": false, "platform": "qts", "processor": "Intel(R) Celeron(R) CPU J3455 @ 1.50GHz", "status": "running", "storageDriver": "overlay2", "uncleanFS": false, "version": { "compose": "1.29.2", "dockerVersion": "20.10.3", "firmware": "4.5.4", "lxc": "2.0.11-1", "lxd": "4.14", "qpkg": "2.4.0.2316", "qpkgBuildDate": "2021/07/06" } }
-
GET
/api/v1/system/resource
¶ Response JSON Object: - cpu_usage (string) – CPU usage in percentage
- memory_usage (object) – Memory usage in MB
Example request
$ curl -sq -b cookies.txt http://${QIP}:${QPORT}/container-station/api/v1/system/resource
Example response
{ "cpu_usage": "100.0", "memory_usage": { "buffers": 585, "cached": 3336, "percent": 26, "percent_buffers": 7, "percent_cached": 42, "total": 7810, "used": 2086 } }
-
GET
/api/v1/system/report
¶ System diagnosis report
Example request
$ curl -sq -b cookies.txt http://${QIP}:${QPORT}/container-station/api/v1/system/report
Network¶
-
GET
/api/v1/system/port/
(string: protocol)/
(string: port)¶ Check port in used or not.
Parameters: - protocol –
tcp
,udp
- port – Port number.
Response JSON Object: - used (boolean) – The port has been used or not.
Example request
$ curl -sq -b cookies.txt http://${QIP}:${QPORT}/container-station/api/v1/system/port/tcp/5000 $ curl -sq -b cookies.txt http://${QIP}:${QPORT}/container-station/api/v1/system/port/udp/33806
Example response
{ "used": true } { "used": false }
- protocol –
-
GET
/api/v1/system/bridge
¶ Get brief bridge information.
Response JSON Object: - interface (string) – Network interface
- ip (string) – IP address
- vswitchName (string) – Virtual switch name
- disabled (boolean) – If it is
true
, do not use this interface - join (boolean) – If it is
true
, means it will join this bridge which was created by others
Example request
$ curl -sq -b cookies.txt http://${QIP}:${QPORT}/container-station/api/v1/system/bridge
Example response
{ "data": [ { "bridge": null, "display": "Adapter 1", "gateway": "192.168.180.254", "ip": "192.168.180.68", "name": "eth0", "netmask": "255.255.254.0" }, { "bridge": null, "display": "Adapter 2", "gateway": "192.168.180.254", "ip": "192.168.181.160", "name": "eth1", "netmask": "255.255.254.0" } ], "default": 1 }
Certificate file¶
-
GET
/api/v1/tls
¶ Get certificate information
Response JSON Object: - startdate (string) – After date
- enddate (string) – Before date
- outdatedNotify (string) – Notify to user
Example request
$ curl -sq -XGET -b cookies.txt http://${QIP}:${QPORT}/container-station/api/v1/tls
Example response
{ "enddate": "2024-07-26", "outdatedNotify": false, "startdate": "2021-08-11" }
-
POST
/api/v1/tls
¶ Set certificate outdated notify
Request JSON Object: - outdatedNotify (boolean) – set to false if not notify next time [required]
Example request
$ curl -sq -XPOST -b cookies.txt -d '{"outdatedNotify": false}' \ http://${QIP}:${QPORT}/container-station/api/v1/tls
Example response
{ "result": true }
-
GET
/api/v1/tls/domain_names
¶ Get extra DNS hostname or IP Address for server certificate
Response JSON Object: - domain_names (string) – Extra hostnames
Example request
$ curl -sq -XGET -b cookies.txt http://${QIP}:${QPORT}/container-station/api/v1/tls/domain_names
Example response
{ "domain_names": "" }
-
PUT
/api/v1/tls/domain_names
¶ Set extra DNS hostname or IP Address for server certificate
Request JSON Object: - domain_names (string) – Extra hostnames [required]
Example request
$ curl -sq -XPUT -b cookies.txt -d '{"domain_names": "user.myqnapcloud.com"}' \ http://${QIP}:${QPORT}/container-station/api/v1/tls/domain_names
Example response
{ "result": true }
-
GET
/api/v1/tls/export
¶ Export certificate files to ZIP format.
Example request
$ curl -sq -XGET -b cookies.txt http://${QIP}:${QPORT}/container-station/api/v1/tls/export
-
POST
/api/v1/tls/rotation
¶ Renew Docker client certificate.
Example request
$ curl -sq -XPOST -b cookies.txt http://${QIP}:${QPORT}/container-station/api/v1/tls/rotation